Custom ArrayList<E>
3 Different ways to convert arrays to Arraylist in java?
Marker interface in Java
ConcurrentHashMap
Feature Name | Description |
---|---|
Lambda expression | A function that can be shared or referred to as an object. |
Functional Interfaces | Single abstract method interface. |
Method References | Uses function as a parameter to invoke a method. |
Default method | It provides an implementation of methods within interfaces enabling 'Interface evolution' facilities. |
Stream API | Abstract layer that provides pipeline processing of the data. |
Date Time API | New improved joda-time inspired APIs to overcome the drawbacks in previous versions |
Optional | Wrapper class to check the null values and helps in further processing based on the value. |
Nashorn, JavaScript Engine | An improvised version of JavaScript Engine that enables JavaScript executions in Java, to replace Rhino. |
Consumer Method Example
What are the significant advantages of Java 8?
- Compact, readable, and reusable code.
- Less boilerplate code.
- Parallel operations and execution.
- Can be ported across operating systems.
- High stability.
- Stable environment.
- Adequate support
What is MetaSpace? How does it differ from PermGen?
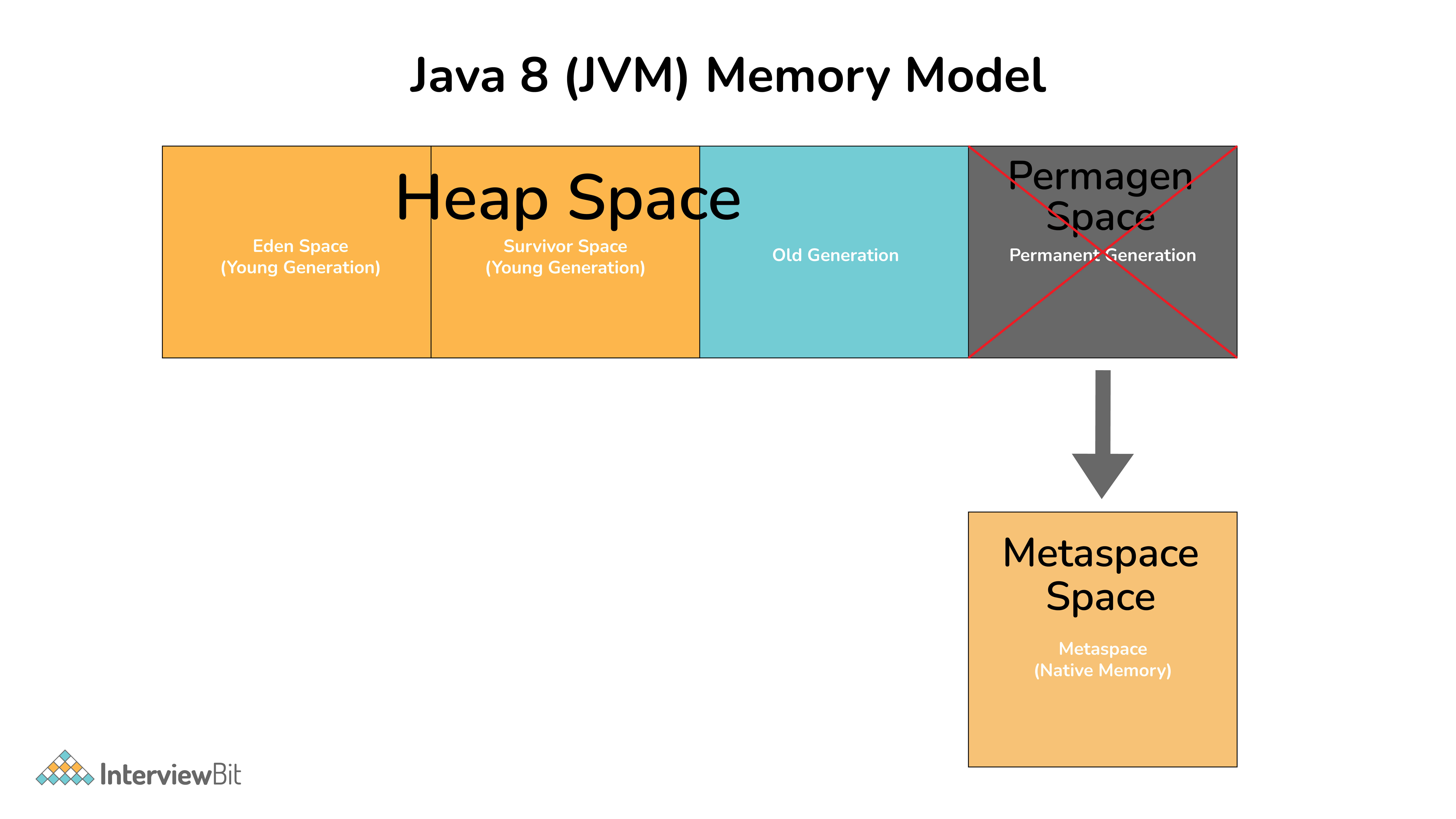
PremGen: MetaData information of classes was stored in PremGen (Permanent-Generation) memory type before Java 8. PremGen is fixed in size and cannot be dynamically resized. It was a contiguous Java Heap Memory.
MetaSpace: Java 8 stores the MetaData of classes in native memory called 'MetaSpace'. It is not a contiguous Heap Memory and hence can be grown dynamically which helps to overcome the size constraints. This improves the garbage collection, auto-tuning, and de-allocation of metadata.
Can a functional interface extend/inherit another interface?
A functional interface cannot extend another interface with abstract methods as it will void the rule of one abstract method per functional interface. E.g:
interface Parent {
public int parentMethod();
}
@FunctionalInterface // This cannot be FunctionalInterface
interface Child extends Parent {
public int childMethod();
// It will also extend the abstract method of the Parent Interface
// Hence it will have more than one abstract method
// And will give a compiler error
}
It can extend other interfaces which do not have any abstract method and only have the default, static, another class is overridden, and normal methods. For eg:
interface Parent {
public void parentMethod(){
System.out.println("Hello");
}
}
@FunctionalInterface
interface Child extends Parent {
public int childMethod();
}